2 - Getting a Codecov account and uploading coverage
Create a new branch step2
git checkout main
git pull
git checkout -b 'step2'
Before we continue, let’s create an account and set up our repository on Codecov.
Create a Codecov account
Create an account on Codecov by clicking on our signup page and following the prompts.
Install the GitHub App Integration
The GitHub app integration is what you will use to determine what repositories that Codecov will have the ability to communicate with and show coverage metrics within the Codecov UI.
Install the Codecov GitHub app integration for the demo repository.
When you are configuring the integration, you have the option to allow access for "All Repositories" or "Only Select Repositories". If you select "Only Select Repositories", be sure to include the demo repository.
Add the Codecov Token to your repo or your org
For more information on tokens, see the documentation.
For the purposes of this tutorial, navigate to the general tab of your repository's configuration page in the Codecov UI. There should be a Repository upload token listed. You'll need to add this token as a variable in your repository settings on GitHub, so copy the token value.
. Note that if the repo has never been used with Codecov, the Repository Upload Token will also be seen on the onboarding page when you click your repo.
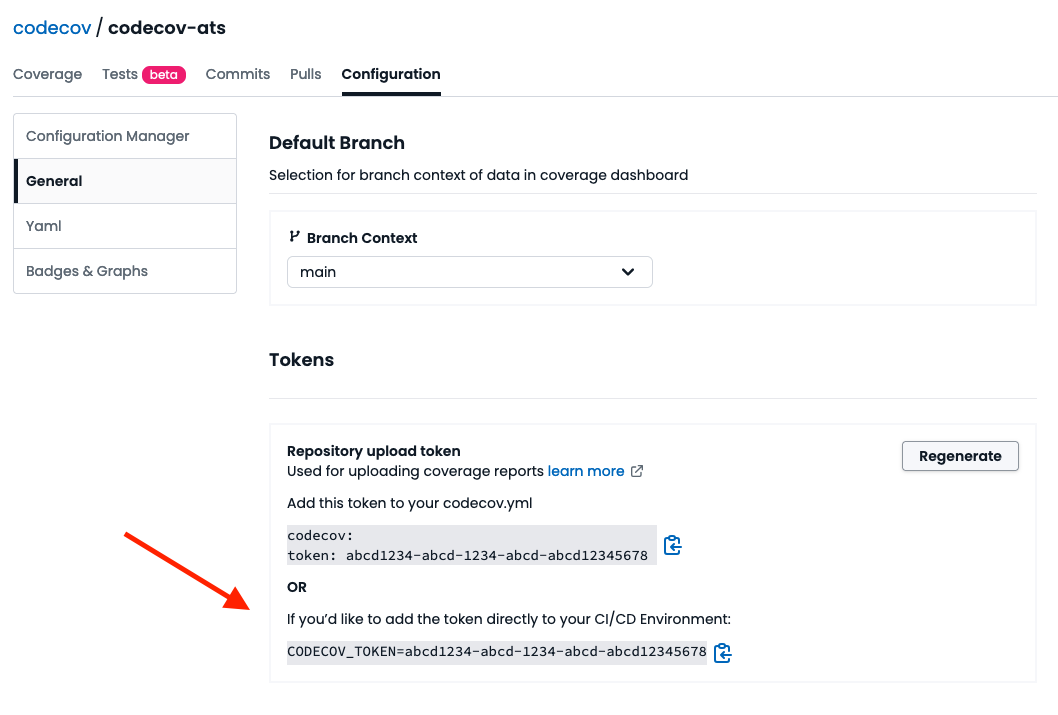
Then, head over to your repository on Github and locate Secret and Variables
under Settings
Finally, paste the token as a new repository secret. Let's call it CODECOV_TOKEN
Create CI pipeline
Create a configuration file for your CI. The CI workflows below will checkout the code, install requirements, run tests, and upload the coverage reports to Codecov.
GitHub Actions
name: API workflow
on: [push, pull_request]
jobs:
build:
runs-on: ubuntu-latest
name: Test python API
steps:
- uses: actions/checkout@v4
- uses: actions/setup-python@v2
with:
python-version: '3.10'
- name: Install requirements
run: pip install -r api/requirements.txt
- name: Run tests and collect coverage
run: pytest --cov=api.calculator --cov-report=xml
- name: Upload coverage reports to Codecov with GitHub Action
uses: codecov/codecov-action@v5
env:
CODECOV_TOKEN: ${{ secrets.CODECOV_TOKEN }}
The upload step uses the Codecov GitHub action. It is built on top of our CLI. It is recommended to use the action
as it automatically runs integrity checks on the binary.
CircleCI
version: 2.1
orbs:
codecov: codecov/[email protected]
jobs:
test-api:
docker:
- image: cimg/python:3.10.2
steps:
- checkout
- run:
name: Install requirements
command: pip install -r api/requirements.txt
- run:
name: Run tests and collect coverage
command: pytest --cov api.calculator
- codecov/upload
workflows:
version: 2.1
build-test:
jobs:
- test-api
The upload step uses the Codecov CircleCI Orb. It is built on top of our CLI. It is recommended to use the Orb
as it automatically runs integrity checks on the binary.
Commit the changes and run the CI workflow
Let’s commit our code and open a pull request. Run
git add .
git commit -m 'step2: upload coverage reports to Codecov'
git push origin step2
Next, go to the demo repository on GitHub and open a pull request (PR).
When opening pull requests, be sure to select your own repository as the base branch.
You will see status checks on the PR from the CI. You can click on them to see the progress of the CI/CD. Once complete, you will receive status checks and a comment from Codecov.
codecov/patch
and codecov/project
status checks
One of Codecov’s core uses is getting code coverage information directly in a developer’s workflow. The status checks shown above are part of that.
- The
project
check is used to maintain a coverage percentage across an entire codebase - The
patch
check is used for code changed in a PR.
A more mature repository may have a strict project
percentage, while a project new to code coverage may only expect new code to be well-tested (patch
coverage). We will be setting some of these values in this section, but you can read more about status checks here.
If you go to the project on Codecov, you will notice that the dashboard is blank. This is because no coverage information has been uploaded onto the default branch. We can fix this by merging the pull request.
Merge the pull request on GitHub and wait for the CI/CD to complete.
When opening pull requests, be sure to select your own repository as the base branch.
Updated 7 months ago